JavaScript Hello World
Last updated onIf you are ready to take your first steps into the realm of web development, then there cannot be a better way other than writing a ‘JavaScript Hello World’ program as shown below.
This article will walk you through the process of building the classic “Hello World!” in JavaScript program.
So, let’s see different ways to write a —”Hello World” program in Javascript:-
- Display the ‘Hello World’ program through the browser console
- Show the “hello world” inside the alert popup box.
- Display the “hello world” within the HTML DOM element.
Before we get started let’s set up our workspace for JavaScript.
Setting Up Your Environment
You only need a text editor and a web browser to begin. Open your preferred text editor—whether it’s Visual Studio Code, Sublime Text, or another—and create a new file. Give it a name like helloWorld.html
to indicate it’s an HTML file.
Start writing your code according to the structure you need, whether it is embedded in JavaScript or an external file.
In this example, we will use embedded JavaScript code within HTML.
<!-- helloWorld.html -->
<!DOCTYPE html>
<html>
<head>
<title>Hello World Program</title>
</head>
<body>
<h1>Welcome to JavaScript Hello World program</h1>
<!-- The embedded JavaScript code would be here -->
<script type="text/javascript">
// => Your JavaScript Here
</script>
</body>
</html>
According to the above HTML structure, we will write our JavaScript code inside the <script>
tag.
Running JavaScript in the Browser: Print “Hello World” in Browser Console
In this program, we will use the console.log
function to print the text in the browser console.
Here is the code of JS.
<!-- helloWorld.html -->
<!DOCTYPE html>
<html>
<head>
<title>Hello World Program</title>
</head>
<body>
<h1>Welcome to JavaScript Hello World program</h1>
<!-- The embedded JavaScript code would be here -->
<script type="text/javascript">
console.log('Hello, World!');
</script>
</body>
</html>
Now, let’s open the browser console by doing the following steps:-
- Right-click on the web page.
- Select the “inspect” object from the menu.
- Navigate to the “Console” tab.
- The output of this program should be printed in the browser console like in the below image.
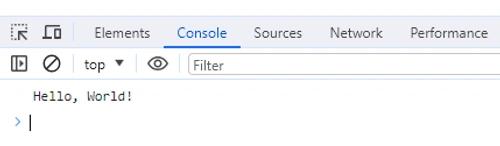
We can also display the same output using JavaScript’s built-in alert
function. This is done through a pop-up box that appears on the webpage during actions.
So, let’s see how can we do that in the following section.
Displaying the Message Using the Alert Function in JavaScript
Basically, JavaScript provides us with a simple notification or message to the user while exploring the browser—It is aleret()
.
Here is an example of its syntax.
alert('Hello, World!');
Let’s see what happens if we add this line of code to our webpage.
<!-- helloWorld.html -->
<!DOCTYPE html>
<html>
<head>
<title>Hello World Program</title>
</head>
<body>
<h1>Welcome to JavaScript Hello World program</h1>
<!-- The embedded JavaScript code would be here -->
<script type="text/javascript">
alert('Hello, World!');
</script>
</body>
</html>
So, when the webpage is loaded the JavaScript will trigger a pop-up on the browser containing a message of — “Hello, World” as a dialog box.
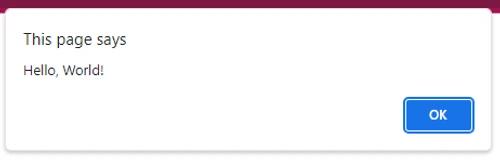
Additionally, there is another way to show — the “Hello, World” text on the webpage using the document.write
. Let’s move on to the following part to understand how can we do that.
Using document.write to Print — the “Hello World” in JavaScript
Actually, document.write
is a built-in method in JavaScript that allows us to write content directly to the webpage.
This function is executed when the browser starts parsing the HTML. Here is an example.
<script type="text/javascript">
document.write('Hello, World!');
</script>
FAQs
What are the three different ways to display a “Hello World” message using JavaScript as mentioned in the article?
You can print the “Hello World” message in three ways in the browser using JavaScript:
- Using the
console.log()
and this will show the text in the browser console. - You can do that using the
alert()
function that shows the pop-up once the webpage is loaded. - Using the
console.write()
and here this method executes the text once the browser parses HTML directly.
How do you use the console.log function to print “Hello World” in the browser console?
To use the console.log()
function, you just have to write the following syntax in your JavaScript block like the below:
<script type="text/javascript">
console.log('Hello, World!');
</script>
So, when the webpage is loaded, the message “Hello, World!” will be printed in the browser console.
What steps should be followed to view the output of the “Hello World” program in the browser console?
To expose the browser console you just need to follow the below steps:-
- Right-click on the web page and select “Inspect” from the menu.
- Click on the console tab in the developer tool.
- When you use
console
object with its functions, the result should appear in the browser console.
What is the purpose of the alert() function in JavaScript, and how is it used to display a “Hello World” message?
The alert()
a pop-up shows a dialog containing a message or notification when the page is loaded.
The syntax alert()
should be like the below:
<script type="text/javascript">
alert('Hello, World!');
</script>
How does the document.write() method work in JavaScript, and when is it executed by the browser?
The console.write()
function writes the content directly into the HTML document. Here is its syntax:
<script type="text/javascript">
document.write('Hello, World!');
</script>
Anyway, let’s summarize it.
Wrapping Up
In this tutorial, you understood how to write a simple JavaScript program — “Hello World” in three methods.
- console.log()
- alert()
- document.write()
Each one of them has a different action to show the content in the browser. Here are the basic examples we already used to expose that.
- The first example uses the console. log() function in order to print the message on the browser console, a quite simplistic and prevalent way of inputting data during the development phase.
- The second example introduces the alert() function, which displays a pop-up modal on your browser and shows a “Hello, World!” message in a dialog box. This is the most common case.
- In the final example, we used the document.write() method to write the “Hello, World!” text to the HTML document.
Thank you for reading. Happy Coding!
Did you find this tutorial useful?
Your feedback helps us improve our tutorials.