Git Pull Force
Last updated onIf you've worked on any coding project, you would know this frustrating wall where Git simply refuses to pull the changes because your local files are different. Fear not, young Padawan; you are not alone. Perhaps you just want to update your codebase without needing to deal with a million merge conflicts. That is where git pull --force
comes in. But hold up—before mashing those keys, you need to be aware that the command has several risks.
In this tutorial, I'm literally going to walk you through everything you need to know about forcing a pull in Git—from why you'd need to do it, to how to do it. Safely. By the time you finish reading this, you'll know how to use git pull --force
without losing your local changes or your mind.
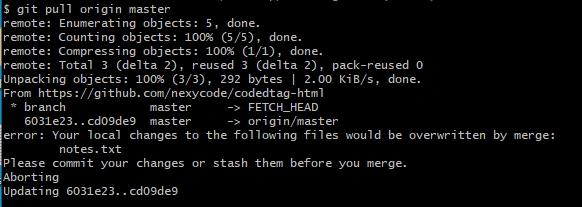
So, let's start with the first method in the next section.
Method 1: Using "git pull --force"
Think of Git as the record keeper of all the changes to your code. When you use git pull
, you're downloading the most updated changes—aka commits—of the remote version of your project and adding that into your version of the project on your computer. But sometimes, your local version has changes that Git hasn't saved yet, and Git doesn't like that mix. That's when you get stuck.
Using git pull --force
is like saying, "I don't care about any of this—just give me the latest version and get rid of anything in the way." You're telling Git to update your local files with whatever is on the remote when you invoke that command; it has no questions.
git pull --force
But here is the catch: it erases your local changes-like, lose-your-homework-before-you-turn-it-in kind of thing. So, be very sure that this is what you want to do.
Anyway, in the following section, you will learn to do that with another method.
Method 2: Using "git fetch" and "git reset" to Force Pull
A less dirty way of forcing a pull in Git uses git fetch
in conjunction with git reset
. This updates your local repository to reflect the one from remote and discards changes that aren't committed as of yet.
Here are the steps:-
1- Fetch the latest changes from the remote
git fetch --all
This will fetch all the latest changes from the remote repository and make updates in your remote-tracking branches without touching your local files.
2- Reset your local branch to match the remote
git reset --hard origin/main
Replace origin/main
with whatever branch you're working on. It resets your local branch to look just like the remote, erasing any changes you haven't committed.
Warning: this will delete all of your local changes that haven't been saved, so make sure you're okay with losing them.
Method 3: Using "git stash" to Temporarily Save Your Changes
If you aren't ready to throw away your local changes but still need to pull the latest from the remote, you can use git stash
. This will temporarily save your changes so that you can pull the new code and then put your changes back.
Here’s how to do it:-
1- Stash your uncommitted changes
git stash
This command stashes your local changes onto a temporary stash and resets your working directory to the last commit.
2- Pull the latest changes from the remote
git pull
Now that there are no conflicts with your working directory, Git can pull changes from the remote without issues.
3- Reapply your stashed changes
git stash apply
Then, after pulling the latest code, you can reapply those saved changes. If there are no conflicts, everything will merge smoothly. If there are conflicts, Git will let you know which files need fixing.
There's also another way using --rebase
. Let's move on to the next section to learn it.
Method 4: Using "git pull --rebase"
While Git has no --force
option for pull, doing a git pull --rebase
will give you a similar result, reapplying your local changes on top of the latest updates from the remote. This is what you want to do in most cases where a straight git pull
would fail; you want to keep your own changes but bring in updated code from the remote.
Here’s how it works:
1- Pull and reapply your changes on top of the latest remote updates:
git pull --rebase
This command fetches the latest changes from the remote and applies your local changes on top of them. A neater approach to handle merging as compared to using the typical merge approach.
2- Handle any conflicts
If there are conflicts, Git will stop the rebase and allow you an opportunity to fix them. Once you have fixed the conflicts, restart the rebase using:
git rebase --continue
Precautions When Using ‘git pull –force’ and ‘git reset’
You can lose your local changes permanently with commands like git pull --force
and git reset
if you are not attentive.
Be safe: before running these commands, you can make a copy of your project or run a command like git stash
to save your changes locally.
Here’s how you can create a backup:
cp -R my-repo my-repo-backup
Or, if you want to stash your changes:
git add .
git commit -m "Temporarily saving changes"
git stash
Once you’ve used
or git pull --force
, you can reapply your stashed changes like this:git reset
Wrapping Up
Learning such powerful commands, like git pull --force
and git reset
, is key to mastering team projects and keeping code in sync. With great power comes great responsibility, so always be very careful with forceful commands and back up your work in case you are ready to lose anything that hasn't been saved. If you keep doing these steps, you are going to be sure to keep your Git workflow running smoothly and easily without stress.
Frequently Asked Questions (FAQs)
What does `git pull --force` actually do?
Will `git pull --force` delete my local changes?
Can I undo `git pull --force` after I run it?
How do I save my changes before running `git pull --force`?
Is there a safer alternative to `git pull --force`?
What’s the difference between `git pull --force` and `git fetch`?
How do I resolve conflicts when using `git pull --rebase`?
What happens if I run `git reset --hard` without committing my changes?
When should I use `git pull --force`?
How can I back up my work before using `git pull --force`?