Classes and objects are important parts of OOP in PHP. They help you organize your code so you can update and expand it more easily. Here, you will see a class and an object with a real example in PHP.
In this article, we will cover the following topics:
- What a class is and how it works.
- What an object is and how to create an instance from a class in PHP.
- Examples.
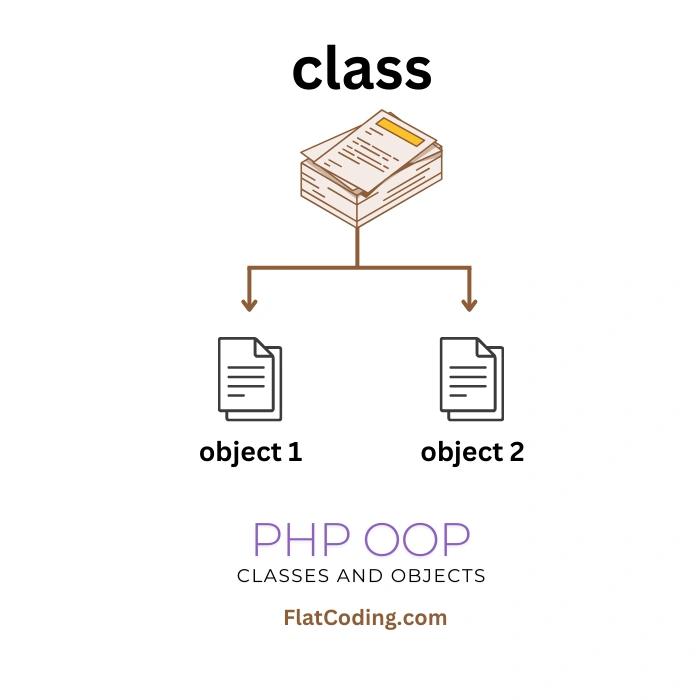
Let’s start with the definition and purposes.
What is a Class in PHP?
A class in PHP is a template that helps developers create objects. It defines variables (called properties) and functions (called methods) that the objects will use.
Properties may be static and should have access modifiers to secure their data. Methods should also have access modifiers and may be static.
Here is an example:
class Languages {
public $name;
public $country;
public function setName($n) {
$this->name= $n;
}
public function setCountry($c){
$this->country= $c;
}
}
This class is called Languages
. The code includes two variables: $name
for the language name and $country
for the country where people speak it.
It also contains two functions. The setName()
function saves a language name, and the setCountry()
function saves a country name.
You can use these functions to set values for the object’s variables when you create an object from this class.
Let’s move on to the following section to see how to get an instance of this class.
What is an Object in PHP?
An object in PHP is an instance that originates from a class. The class is the plan, and the object is the real thing you can use. Each object has its own data and can use the functions from the class.
We will proceed with the previous example. Let’s create an instance of the Languages class.
$lang = new Languages();
// Add the language
$lang->setName("Japanese");
// Add the country name
$lang->setCountry("Japan");
// Print result
echo "{$lang->country} is in Asia, and their mother tongue is {$lang->name}.";
The output:
Japan is in Asia, and their mother tongue is Japanese.
Here is the full example:
class Languages {
public $name;
public $country;
public function setName($n) {
$this->name= $n;
}
public function setCountry($c){
$this->country= $c;
}
}
$lang = new Languages();
$lang->setName("Japanese");
$lang->setCountry("Japan");
echo "{$lang->country} is in Asia, and their mother tongue is {$lang->name}.";
Let’s see more examples:
Examples of Class and Object in PHP
Example 1: This class stores the name of a sport and the number of players required.
class Sport {
public $name;
public $players;
public function setSport($n, $p) {
$this->name = $n;
$this->players = $p;
}
public function getSportInfo() {
return "The sport {$this->name} needs {$this->players} players.";
}
}
$sport1 = new Sport();
$sport1->setSport("Football", 11);
echo $sport1->getSportInfo();
Here is the output:
The sport Football needs 11 players.
How it works:
- The class Sport defines two variables:
$name
stores the sport’s name.$players
stores the number of players.
- The setSport() method assigns values to these variables.
- It takes two inputs:
$n
for the name and$p
for the number of players. - It stores them in the class properties
$name
and$players
.
- It takes two inputs:
- The getSportInfo() method returns a sentence with the sport’s details.
Example 2: This stores clothing type and color in a class.
class Fashion {
public $type;
public $color;
public function setClothing($t, $c) {
$this->type = $t;
$this->color = $c;
}
public function getClothingInfo() {
return "This {$this->color} {$this->type} looks stylish.";
}
}
$shirt = new Fashion();
$shirt->setClothing("shirt", "blue");
echo $shirt->getClothingInfo();
The output:
This blue shirt looks stylish.
The Fashion class has two properties: $type
for the clothing type and $color
for its color.
The setClothing()
method takes two inputs, $t
for type and $c
for color, then assigns them to the class properties.
The getClothingInfo()
method returns a sentence that describes the clothing item.
Example 3: This class stores a natural element and its description.
class Nature {
public $element;
public $description;
public function setElement($e, $d) {
$this->element = $e;
$this->description = $d;
}
public function getElementInfo() {
return "{$this->element} is {$this->description}.";
}
}
$sea = new Nature();
$sea->setElement("Sea", "vast and deep");
echo $sea->getElementInfo();
Here is the output:
Sea is vast and deep.
The Nature class stores information about natural elements. It has two properties: $element
, which holds the name (e.g., sea, earth), and $description
, which describes it.
The setElement() method assigns values to these properties with take two inputs: $e
for the element name and $d
for its description.
It then stores them in $element
and $description
. The getElementInfo() method returns a sentence describing the element.
Wrapping Up
You learned how to use a class and an object with a real example in OOP PHP. Here is a quick recap:
A class is a template that defines variables (properties) and functions (methods). An object is a real instance of a class that holds data and performs actions using the class’s methods.
Key Takeaways:
- A class is a group of related properties and methods.
- An object comes from a class and stores its own values.
- You create an object with the
new
keyword. - Objects can call methods to set and get data.
FAQ’s
Why use a class in PHP?
What is a class and object with an example?
class Animal {
public $type;
public function setName($n) {
$this->type = $n;
}
}
$myAnimal = new Animal();
$myAnimal->setName("Lion");
Here, Animal is the class and $myAnimal is an object.